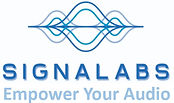
DC removal
What is your task?
We received a PDM file (a text file containing 1 and -1 values) and, using a CIC filter, convert it to a PCM file. Finally, we convert it to a WAV file and return it as output.
How does the code you wrote solve the problem? What is the algorithm?
The code is designed to convert a digital pulse signal in PDM format to PCM format and save it as a WAV file. The main algorithm is as follows:
1. **Reading from PDM File**: The `read_pdm_file` function reads the PDM signal from a text file where each line represents a value of -1 or 1.
2. **CIC Filter**: The `cic_filter` function implements a CIC filter to process the PDM signal. The filter consists of three stages:
- **Integration Stage**: Cumulative summation of the PDM signal.
- **Decimation**: Reducing the sampling rate by selecting every n-th sample.
- **Combination Stage**: Calculating the difference between successive samples in the decimation stage output.
3. **Converting PCM to WAV**: The `save_pcm_as_wav` function takes the processed PCM signal and converts it to WAV format. It scales the signal to the int16 range, provides the WAV file syntax, and saves the file to a chosen location.
What were the challenges that required the use of existing modules and what do these modules do?
We used NumPy because it provides advanced numerical data types like arrays, which allow for faster processing and computation compared to standard Python lists. This facilitates handling large datasets efficiently.
We also used the `scipy.io.wavfile.write` function to enable saving a WAV file from the PCM signal generated by the code. The SciPy library offers rich tools for data processing and analysis, including tools for working with audio files, such as conversion and saving WAV files. The `write` function from `scipy.io.wavfile` allows for creating a WAV file from PCM data in various formats, setting the sampling rate, and saving the audio efficiently and conveniently.
What types of inputs does the code accept and what should be expected in the output?
The code accepts a text file as input. The output should be a WAV file, which is an audio file.
Explain the parameter names in the code and their roles:
- pdm_file_path:
Type: String (str)
Description: Path to the text file containing PDM signal data. The PDM signal contains values of -1 and 1.
- wav_file_path:
Type: String (str)
Description: Path to where the generated WAV file will be saved. This includes the file name and the .wav extension.
- sample_rate:
Type: Integer (int)
Description: Desired sampling rate for the WAV file, specified in Hertz (Hz). The sampling rate indicates how many audio data points are captured per second. Higher sampling rates generally ensure better sound quality.
- decimation_factor:
Type: Integer (int)
Default: 32
Description: Decimation factor used in the CIC (Cascaded Integrator-Comb) filter. Decimation reduces the sample rate of the signal by an integer factor. In this context, it determines how many samples are taken from the integrator output for each filter output.
- pdm_signal:
Type: NumPy Array (np.array)
Description: Array containing the PDM signal read from the input file. The PDM signal represents audio data where each sample is either -1 or 1.
- pcm_signal:
Type: NumPy Array (np.array)
Description: Array containing the filtered PCM (Pulse Code Modulation) signal obtained from the CIC filter applied to the PDM signal.
- normalized_signal:
Type: NumPy Array (np.array)
Description: The filtered PCM signal scaled to the range of -1 to 1. Normalization ensures the signal fits the dynamic range required for conversion to 16-bit PCM format.
- scaled_signal:
Type: NumPy Array (np.array)
Description: The normalized PCM signal scaled to 16-bit integer values ranging from -32767 to 32767.
- int16_signal:
Type: NumPy Array (np.array)
Description: The final output of the PCM signal represented as a single array of 16-bit integers (int16). This format is required by the `write` function from `scipy.io.wavfile` to save the data in a WAV file.
Section Title
Section Subtitle
Every website has a story, and your visitors want to hear yours. This space is a great opportunity to give a full background on who you are, what your team does and what your site has to offer. Double click on the text box to start editing your content and make sure to add all the relevant details you want site visitors to know.
If you’re a business, talk about how you started and share your professional journey. Explain your core values, your commitment to customers and how you stand out from the crowd. Add a photo, gallery or video for even more engagement.