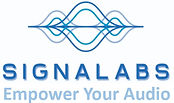
DC removal
Expand on the task you have been given. What is the problem you are trying to solve?
The task is to implement a noise reduction filter in the time-frequency domain using spectral subtraction. Spectral subtraction aims to recover the power or magnitude of a signal that has been corrupted by noise. Signals we transmit or record often come with noise that complicates extracting the original information. The overall approach involves using 0.25 seconds of audio as a noise sample to compute the noise spectrum, which is then subtracted from the segments identified as noisy. Spectral subtraction is an effective solution when the signal cannot be separated from the noise and the noise distorts the signal.
How does the code you wrote solve the problem?
The algorithm follows these general steps:
1. Load a WAV file and normalize it to values in the range [-1,1].
2. Extract the first 0.25 seconds of audio to sample the noise.
3. Apply Short-Time Fourier Transform (STFT) to this noise sample to move to the frequency domain.
4. Compute the noise spectrum (main magnitude) to be used for noise reduction.
5. Apply STFT to the entire signal, obtaining magnitude and phase for each frame.
6. Subtract the noise magnitude from each magnitude calculated, which reduces the noise.
7. Set negative values to 0.
8. Combine the magnitudes with the original phases in the frequency domain and convert back to the time domain using Inverse STFT (ISTFT).
9. Normalize the signal to fit within a 16-bit range.
10. Convert the result to a WAV file.
What do these modules do?
SciPy: Used for reading and saving WAV files.
NumPy: Used for numerical operations, such as handling arrays and performing calculations efficiently.
What types of inputs does the code accept and what should be expected in the output?
Input: A WAV signal with constant noise.
Output: A WAV signal with reduced noise.
Explain the parameter names in the code and their roles:
- input_file:
Type: String (str)
Description: Path to the file containing the noisy audio signal (input).
- output_file:
Type: String (str)
Description: Path to where the processed audio signal will be saved, including the file name and the .wav extension.
- sample_rate:
Type: Integer (int)
Description: Sampling rate of the audio, specifying how many samples per second are recorded.
- waveform:
Type: Array (NumPy)
Description: The audio signal after normalization.
- stft_matrix:
Type: Matrix (NumPy)
Description: The STFT matrix of the audio signal, representing the time-frequency domain of the signal.
- magnitude_spectrum:
Type: Array (NumPy)
Description: Magnitude of the STFT matrix, representing the strength of frequencies at each time point.
- phase_spectrum:
Type: Array (NumPy)
Description: Phase of the STFT matrix, representing the phase angle of frequencies at each time point.
- complex_spectrum:
Type: Array (NumPy)
Description: Complex matrix built from the phase spectrum, used for reconstructing the signal in the ISTFT.
- frame_size:
Type: Integer (int)
Description: Number of samples in each frame for STFT.
- hop_size:
Type: Integer (int)
Description: Step size or number of samples between the start points of consecutive frames.
- initial_threshold_factor:
Type: Float (float)
Description: Initial factor for the noise energy threshold used to identify noisy frames.
- max_threshold_adjustments:
Type: Integer (int)
Description: Maximum number of adjustments allowed for the energy threshold if no noisy frames are detected initially.
- threshold_adjustments_attempts:
Type: Integer (int)
Description: Number of attempts made to find the energy threshold factor for noisy frames.
- noisy_energy_threshold:
Type: Float (float)
Description: Energy threshold used to classify frames as noisy or not based on their energy.
- noise_frame:
Type: List (NumPy)
Description: List of frames classified as noise based on the energy threshold.
- concatenated_noise_signal:
Type: Array (NumPy)
Description: Concatenated noise signal composed of all classified noise frames.
- noise_stft_matrix:
Type: Matrix (NumPy)
Description: STFT matrix of the concatenated noise signal.
- noise_magnitude_spectrum:
Type: Array (NumPy)
Description: Magnitude spectrum of the noise signal, used for spectral subtraction.
- mean_noise_spectrum:
Type: Array (NumPy)
Description: Average magnitude of the noise spectrum, used for spectral subtraction.
- cleaned_spectrum:
Type: Array (NumPy)
Description: Spectrum of the input signal after subtracting the mean noise spectrum.
- cleaned_complex_spectrum:
Type: Array (NumPy)
Description: Complex spectrum obtained by applying phase information to the cleaned spectrum.
- output_waveform:
Type: Array (NumPy)
Description: Final processed audio signal in the time domain, ready to be saved to the output file.
Section Title
Section Subtitle
Every website has a story, and your visitors want to hear yours. This space is a great opportunity to give a full background on who you are, what your team does and what your site has to offer. Double click on the text box to start editing your content and make sure to add all the relevant details you want site visitors to know.
If you’re a business, talk about how you started and share your professional journey. Explain your core values, your commitment to customers and how you stand out from the crowd. Add a photo, gallery or video for even more engagement.