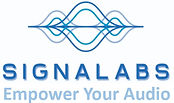
DC removal
What is the problem you came to solve?
The main problem we aimed to solve is the dynamic variability between different segments of audio. Audio files can contain sections where the volume levels vary significantly. This can result in a situation where details are lost or disturbances occur in the audio. For example, if the volume is too high, the signal might distort and cause audio clipping, making it impossible for the system to handle and process it properly.
How does the code you wrote solve the problem?
Steps in the code:
1. Reading a WAV File:
- Use the `wave` module to open the specific WAV file (input_wav) and read the audio data from it.
- Obtain technical information about the file, such as the number of channels, sample resolution, sample rate, etc.
2. Converting Data to Numpy Array:
- Convert the binary data read from the WAV file into a Numpy array of 16-bit integers (np.int16). This enables efficient processing of the audio data in the code.
3. Applying AGC (Automatic Gain Control):
- Calculate the peak amplitude and RMS amplitude of the audio data using mathematical calculations in Numpy.
- Compute the current dBFS of the data to understand how it will appear on the full scale.
4. Gain Adjustment Calculation:
- Calculate the required gain adjustment to match the desired dBFS level. This involves computing the maximum gain limits that can be applied to the audio data to avoid audio clipping or distortion.
5. Applying Gain to Audio Data:
- Apply the calculated gain to the original audio data to achieve the audio data adjusted with AGC to the desired dBFS level.
6. Saving the Processed Audio Data:
- Save the successfully adjusted audio data to a new WAV file (output_wav) using the `wave` module, and set technical parameters like the number of channels, sample resolution, and sample rate.
What challenges required you to use existing modules, and what do the modules do?
1. Format Support and Compatibility:
- Modules like `wave` expect files to be in a proper and compatible format. If the file does not meet the WAV standard, issues such as errors in reading or data transfer might occur.
2. Memory Management and Performance:
- Processing large WAV files or high sample rates may require skilled memory management and performance enhancement. Memory issues can arise when working with large audio data.
3. Precision and Accurate Processing:
- Processing a WAV file often requires high precision and accurate communication between the original audio data and the processed output. This might require advanced solutions for complex audio processing.
Functions of the Mentioned Modules:
- Wave Module:
- Read and Write Functions: Allows reading and writing of WAV files, including safety checks to ensure they conform to the WAV standard.
- File Properties Reading: Allows obtaining information like the number of channels, sample resolution, sample rate, etc.
- Numpy Module:
- Data Arrays: Facilitates fast and efficient processing of data arrays, especially for audio data in various formats.
- Numerical Calculations: Provides advanced mathematical operations like amplification, dynamic concatenation, and data encoding, aiding in the implementation of functions like AGC.
What types of inputs does the code accept, and what should be expected in the output?
- Inputs:
- The code accepts inputs in the form of WAV files. The `read_wav` function expects the name of the WAV file as an argument, reads the audio data from this file, and returns the audio data (in Numpy array format) and the sample rate of the WAV file.
- Expected Output:
- If all operations are successful, a processed WAV file with AGC applied to meet the target RMS level will be saved. A message will be printed indicating that the file has been successfully saved.
Explain the parameter names in the code and their roles:
- Parameters:
- input_wav: Path to the original WAV file you want to process with AGC (Automatic Gain Control). The function attempts to open and read audio data from this file.
- output_wav: Path to the WAV file where the result of the AGC processing will be saved. After processing the audio data, the result will be written to this file.
- target_dbfs: The target loudness level in dBFS (Decibels Full Scale). This parameter specifies the level at which you want the output file to appear after AGC processing. For example, -20 dBFS is a common level for radio broadcasts or streaming.
- max_gain: The maximum allowed gain size to be added to the audio data. This parameter prevents excessive amplification that could lead to audio clipping or distortion.
Variables within the functions:
- wave_file: Variable holding the `wave.open()` object representing the WAV file being read. It provides methods (getnchannels(), getsampwidth(), getframerate(), getnframes(), readframes()) to extract information about the audio file and read its frames.
- num_channels: Variable storing the number of audio channels (e.g., mono or stereo) in the WAV file.
- sample_width: Variable storing the sample width (in bytes) of each audio sample. It indicates the data resolution or bit depth of the audio data.
- frame_rate: Variable storing the sample rate (samples per second) of the WAV file. It specifies how often the audio samples are taken per second.
- num_frames: Variable storing the total number of frames in the WAV file (also referred to as audio samples).
- frames: Variable holding the binary data read from the WAV file, representing the audio samples.
- audio_data: Variable storing the audio data converted from binary to a Numpy array of 16-bit integers. It represents the audio data read from the WAV file.
- audio_data_agc: Variable holding the audio data after AGC processing. It stores the adjusted audio data ready to be written to the output WAV file.
Section Title
Section Subtitle
Every website has a story, and your visitors want to hear yours. This space is a great opportunity to give a full background on who you are, what your team does and what your site has to offer. Double click on the text box to start editing your content and make sure to add all the relevant details you want site visitors to know.
If you’re a business, talk about how you started and share your professional journey. Explain your core values, your commitment to customers and how you stand out from the crowd. Add a photo, gallery or video for even more engagement.